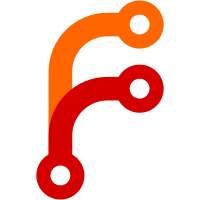
Некоторые проверки провалились
test / test (1.16.x) (push) Has been cancelled
test / test (1.17.x) (push) Has been cancelled
82 строки
2,2 КиБ
Go
82 строки
2,2 КиБ
Go
package builder_test
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"os/exec"
|
|
"path/filepath"
|
|
"testing"
|
|
|
|
"git.golang1.ru/softonik/godog"
|
|
)
|
|
|
|
func testOutsideGopathAndHavingOnlyFeature(t *testing.T) {
|
|
builderTC := builderTestCase{}
|
|
|
|
builderTC.dir = filepath.Join(os.TempDir(), t.Name(), "godogs")
|
|
builderTC.files = map[string]string{
|
|
"godogs.feature": builderFeatureFile,
|
|
}
|
|
|
|
builderTC.goModCmds = make([]*exec.Cmd, 2)
|
|
builderTC.goModCmds[0] = exec.Command("go", "mod", "init", "godogs")
|
|
|
|
godogDependency := fmt.Sprintf("git.golang1.ru/softonik/godog@%s", godog.Version)
|
|
builderTC.goModCmds[1] = exec.Command("go", "mod", "edit", "-require", godogDependency)
|
|
|
|
builderTC.run(t)
|
|
}
|
|
|
|
func testOutsideGopath(t *testing.T) {
|
|
builderTC := builderTestCase{}
|
|
|
|
builderTC.dir = filepath.Join(os.TempDir(), t.Name(), "godogs")
|
|
builderTC.files = map[string]string{
|
|
"godogs.feature": builderFeatureFile,
|
|
"godogs.go": builderMainCodeFile,
|
|
"godogs_test.go": builderTestFile,
|
|
}
|
|
|
|
builderTC.goModCmds = make([]*exec.Cmd, 1)
|
|
builderTC.goModCmds[0] = exec.Command("go", "mod", "init", "godogs")
|
|
|
|
builderTC.run(t)
|
|
}
|
|
|
|
func testOutsideGopathWithXTest(t *testing.T) {
|
|
builderTC := builderTestCase{}
|
|
|
|
builderTC.dir = filepath.Join(os.TempDir(), t.Name(), "godogs")
|
|
builderTC.files = map[string]string{
|
|
"godogs.feature": builderFeatureFile,
|
|
"godogs.go": builderMainCodeFile,
|
|
"godogs_test.go": builderXTestFile,
|
|
}
|
|
|
|
builderTC.goModCmds = make([]*exec.Cmd, 1)
|
|
builderTC.goModCmds[0] = exec.Command("go", "mod", "init", "godogs")
|
|
|
|
builderTC.run(t)
|
|
}
|
|
|
|
func testInsideGopath(t *testing.T) {
|
|
builderTC := builderTestCase{}
|
|
|
|
gopath := filepath.Join(os.TempDir(), t.Name(), "_gp")
|
|
defer os.RemoveAll(gopath)
|
|
|
|
builderTC.dir = filepath.Join(gopath, "src", "godogs")
|
|
builderTC.files = map[string]string{
|
|
"godogs.feature": builderFeatureFile,
|
|
"godogs.go": builderMainCodeFile,
|
|
"godogs_test.go": builderTestFile,
|
|
}
|
|
|
|
builderTC.goModCmds = make([]*exec.Cmd, 1)
|
|
builderTC.goModCmds[0] = exec.Command("go", "mod", "init", "godogs")
|
|
builderTC.goModCmds[0].Env = os.Environ()
|
|
builderTC.goModCmds[0].Env = append(builderTC.goModCmds[0].Env, "GOPATH="+gopath)
|
|
builderTC.goModCmds[0].Env = append(builderTC.goModCmds[0].Env, "GO111MODULE=on")
|
|
|
|
builderTC.run(t)
|
|
}
|