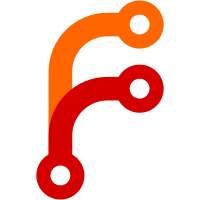
This PR fixes two bugs at once: 1. Indices were incorrectly extended to a bigger type. Specifically, unsigned integers were sign extended and signed integers were zero extended. This commit swaps them around. 2. The getelementptr instruction was given the raw index, even if it was a uint8 for example. However, getelementptr assumes the indices are signed, and therefore an index of uint8(200) was interpreted as an index of int8(-56).
34 строки
546 Б
Go
34 строки
546 Б
Go
package main
|
|
|
|
func someString() string {
|
|
return "foo"
|
|
}
|
|
|
|
func zeroLengthString() string {
|
|
return ""
|
|
}
|
|
|
|
func stringLen(s string) int {
|
|
return len(s)
|
|
}
|
|
|
|
func stringIndex(s string, index int) byte {
|
|
return s[index]
|
|
}
|
|
|
|
func stringCompareEqual(s1, s2 string) bool {
|
|
return s1 == s2
|
|
}
|
|
|
|
func stringCompareUnequal(s1, s2 string) bool {
|
|
return s1 != s2
|
|
}
|
|
|
|
func stringCompareLarger(s1, s2 string) bool {
|
|
return s1 > s2
|
|
}
|
|
|
|
func stringLookup(s string, x uint8) byte {
|
|
// Test that x is correctly extended to an uint before comparison.
|
|
return s[x]
|
|
}
|