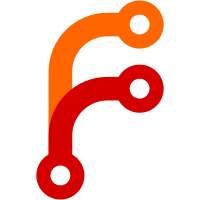
This commit changes the number of wait states for the stm32f103 chip to 2 instead of 4. This gets it back in line with the datasheet, but it also has the side effect of breaking I2C. Therefore, another (seemingly unrelated) change is needed: the i2cTimeout constant must be increased to a higher value to adjust to the lower flash wait states - presumably because the lower number of wait states allows the chip to run code faster.
44 строки
1,1 КиБ
Go
44 строки
1,1 КиБ
Go
// +build stm32f7x2
|
|
|
|
package machine
|
|
|
|
// Peripheral abstraction layer for the stm32f407
|
|
|
|
import (
|
|
"device/stm32"
|
|
"runtime/interrupt"
|
|
)
|
|
|
|
func CPUFrequency() uint32 {
|
|
return 216000000
|
|
}
|
|
|
|
//---------- UART related types and code
|
|
|
|
// UART representation
|
|
type UART struct {
|
|
Buffer *RingBuffer
|
|
Bus *stm32.USART_Type
|
|
Interrupt interrupt.Interrupt
|
|
AltFuncSelector uint8
|
|
}
|
|
|
|
// Configure the UART.
|
|
func (uart UART) configurePins(config UARTConfig) {
|
|
// enable the alternate functions on the TX and RX pins
|
|
config.TX.ConfigureAltFunc(PinConfig{Mode: PinModeUARTTX}, uart.AltFuncSelector)
|
|
config.RX.ConfigureAltFunc(PinConfig{Mode: PinModeUARTRX}, uart.AltFuncSelector)
|
|
}
|
|
|
|
// UART baudrate calc based on the bus and clockspeed
|
|
// NOTE: keep this in sync with the runtime/runtime_stm32f7x2.go clock init code
|
|
func (uart UART) getBaudRateDivisor(baudRate uint32) uint32 {
|
|
var clock uint32
|
|
switch uart.Bus {
|
|
case stm32.USART1, stm32.USART6:
|
|
clock = CPUFrequency() / 2 // APB2 Frequency
|
|
case stm32.USART2, stm32.USART3, stm32.UART4, stm32.UART5:
|
|
clock = CPUFrequency() / 8 // APB1 Frequency
|
|
}
|
|
return clock / baudRate
|
|
}
|