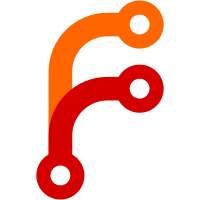
On WebAssembly it is possible to grow the heap with the memory.grow instruction. This commit implements this feature and with that also removes the -heap-size flag that was reportedly broken (I haven't verified that). This should make it easier to use TinyGo for WebAssembly, where there was no good reason to use a fixed heap size. This commit has no effect on baremetal targets with optimizations enabled.
37 строки
745 Б
Go
37 строки
745 Б
Go
// +build gc.none
|
|
|
|
package runtime
|
|
|
|
// This GC strategy provides no memory allocation at all. It can be useful to
|
|
// detect where in a program memory is allocated, or to combine this runtime
|
|
// with a separate (external) garbage collector.
|
|
|
|
import (
|
|
"unsafe"
|
|
)
|
|
|
|
func alloc(size uintptr) unsafe.Pointer
|
|
|
|
func free(ptr unsafe.Pointer) {
|
|
// Nothing to free when nothing gets allocated.
|
|
}
|
|
|
|
func GC() {
|
|
// Unimplemented.
|
|
}
|
|
|
|
func KeepAlive(x interface{}) {
|
|
// Unimplemented. Only required with SetFinalizer().
|
|
}
|
|
|
|
func SetFinalizer(obj interface{}, finalizer interface{}) {
|
|
// Unimplemented.
|
|
}
|
|
|
|
func initHeap() {
|
|
// Nothing to initialize.
|
|
}
|
|
|
|
func setHeapEnd(newHeapEnd uintptr) {
|
|
// Nothing to do here, this function is never actually called.
|
|
}
|