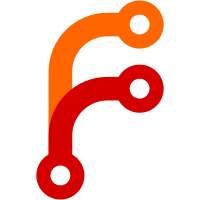
On WebAssembly it is possible to grow the heap with the memory.grow instruction. This commit implements this feature and with that also removes the -heap-size flag that was reportedly broken (I haven't verified that). This should make it easier to use TinyGo for WebAssembly, where there was no good reason to use a fixed heap size. This commit has no effect on baremetal targets with optimizations enabled.
24 строки
635 Б
Go
24 строки
635 Б
Go
// +build darwin linux,!baremetal,!wasi freebsd,!baremetal
|
|
// +build !nintendoswitch
|
|
|
|
// +build gc.conservative gc.leaking
|
|
|
|
package runtime
|
|
|
|
const heapSize = 1 * 1024 * 1024 // 1MB to start
|
|
|
|
var heapStart, heapEnd uintptr
|
|
|
|
func preinit() {
|
|
heapStart = uintptr(malloc(heapSize))
|
|
heapEnd = heapStart + heapSize
|
|
}
|
|
|
|
// growHeap tries to grow the heap size. It returns true if it succeeds, false
|
|
// otherwise.
|
|
func growHeap() bool {
|
|
// At the moment, this is not possible. However it shouldn't be too
|
|
// difficult (at least on Linux) to allocate a large amount of virtual
|
|
// memory at startup that is then slowly used.
|
|
return false
|
|
}
|