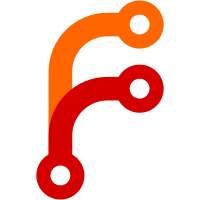
This patch adds a new pragma for functions and globals to set the section name. This can be useful to place a function or global in a special device specific section, for example: * Functions may be placed in RAM to make them run faster, or in flash (if RAM is the default) to not let them take up RAM. * DMA memory may only be placed in a special memory area. * Some RAM may be faster than other RAM, and some globals may be performance critical thus placing them in this special RAM area can help. * Some (large) global variables may need to be placed in external RAM, which can be done by placing them in a special section. To use it, you have to place a function or global in a special section, for example: //go:section .externalram var externalRAMBuffer [1024]byte This can then be placed in a special section of the linker script, for example something like this: .bss.extram (NOLOAD) : { *(.externalram) } > ERAM
66 строки
1,5 КиБ
Go
66 строки
1,5 КиБ
Go
package main
|
|
|
|
import _ "unsafe"
|
|
|
|
// Creates an external global with name extern_global.
|
|
//go:extern extern_global
|
|
var externGlobal [0]byte
|
|
|
|
// Creates a
|
|
//go:align 32
|
|
var alignedGlobal [4]uint32
|
|
|
|
// Test conflicting pragmas (the last one counts).
|
|
//go:align 64
|
|
//go:align 16
|
|
var alignedGlobal16 [4]uint32
|
|
|
|
// Test exported functions.
|
|
//export extern_func
|
|
func externFunc() {
|
|
}
|
|
|
|
// Define a function in a different package using go:linkname.
|
|
//go:linkname withLinkageName1 somepkg.someFunction1
|
|
func withLinkageName1() {
|
|
}
|
|
|
|
// Import a function from a different package using go:linkname.
|
|
//go:linkname withLinkageName2 somepkg.someFunction2
|
|
func withLinkageName2()
|
|
|
|
// Function has an 'inline hint', similar to the inline keyword in C.
|
|
//go:inline
|
|
func inlineFunc() {
|
|
}
|
|
|
|
// Function should never be inlined, equivalent to GCC
|
|
// __attribute__((noinline)).
|
|
//go:noinline
|
|
func noinlineFunc() {
|
|
}
|
|
|
|
// This function should have the specified section.
|
|
//go:section .special_function_section
|
|
func functionInSection() {
|
|
}
|
|
|
|
//export exportedFunctionInSection
|
|
//go:section .special_function_section
|
|
func exportedFunctionInSection() {
|
|
}
|
|
|
|
// This function should not: it's only a declaration and not a definition.
|
|
//go:section .special_function_section
|
|
func undefinedFunctionNotInSection()
|
|
|
|
//go:section .special_global_section
|
|
var globalInSection uint32
|
|
|
|
//go:section .special_global_section
|
|
//go:extern undefinedGlobalNotInSection
|
|
var undefinedGlobalNotInSection uint32
|
|
|
|
//go:align 1024
|
|
//go:section .global_section
|
|
var multipleGlobalPragmas uint32
|