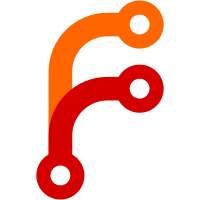
I found that when I enable ThinLTO, a miscompilation triggers that had been hidden all the time previously. The bug appears to happen as follows: 1. TinyGo generates a function with a runtime.trackPointer call, but without an alloca (or the alloca gets optimized away). 2. LLVM sees that no alloca needs to be kept alive across the runtime.trackPointer call, and therefore it adds the 'tail' flag. One of the effects of this flag is that it makes it undefined behavior to keep allocas alive across the call (which is still safe at that point). 3. The GC lowering pass adds a stack slot alloca and converts runtime.trackPointer calls into alloca stores. The last step triggers the bug: the compiler inserts an alloca where there was none before but that's not valid as long as the 'tail' flag is present. This patch fixes the bug in a somewhat dirty way, by always creating a dummy alloca so that LLVM won't do the optimization in step 2 (and possibly other optimizations that rely on there being no alloca instruction).
61 строка
2,1 КиБ
LLVM
61 строка
2,1 КиБ
LLVM
; ModuleID = 'go1.20.go'
|
|
source_filename = "go1.20.go"
|
|
target datalayout = "e-m:e-p:32:32-p10:8:8-p20:8:8-i64:64-n32:64-S128-ni:1:10:20"
|
|
target triple = "wasm32-unknown-wasi"
|
|
|
|
%runtime._string = type { ptr, i32 }
|
|
|
|
declare noalias nonnull ptr @runtime.alloc(i32, ptr, ptr) #0
|
|
|
|
declare void @runtime.trackPointer(ptr nocapture readonly, ptr, ptr) #0
|
|
|
|
; Function Attrs: nounwind
|
|
define hidden void @main.init(ptr %context) unnamed_addr #1 {
|
|
entry:
|
|
ret void
|
|
}
|
|
|
|
; Function Attrs: nounwind
|
|
define hidden ptr @main.unsafeSliceData(ptr %s.data, i32 %s.len, i32 %s.cap, ptr %context) unnamed_addr #1 {
|
|
entry:
|
|
%stackalloc = alloca i8, align 1
|
|
call void @runtime.trackPointer(ptr %s.data, ptr nonnull %stackalloc, ptr undef) #2
|
|
ret ptr %s.data
|
|
}
|
|
|
|
; Function Attrs: nounwind
|
|
define hidden %runtime._string @main.unsafeString(ptr dereferenceable_or_null(1) %ptr, i16 %len, ptr %context) unnamed_addr #1 {
|
|
entry:
|
|
%stackalloc = alloca i8, align 1
|
|
%0 = icmp slt i16 %len, 0
|
|
%1 = icmp eq ptr %ptr, null
|
|
%2 = icmp ne i16 %len, 0
|
|
%3 = and i1 %1, %2
|
|
%4 = or i1 %3, %0
|
|
br i1 %4, label %unsafe.String.throw, label %unsafe.String.next
|
|
|
|
unsafe.String.next: ; preds = %entry
|
|
%5 = zext i16 %len to i32
|
|
%6 = insertvalue %runtime._string undef, ptr %ptr, 0
|
|
%7 = insertvalue %runtime._string %6, i32 %5, 1
|
|
call void @runtime.trackPointer(ptr %ptr, ptr nonnull %stackalloc, ptr undef) #2
|
|
ret %runtime._string %7
|
|
|
|
unsafe.String.throw: ; preds = %entry
|
|
call void @runtime.unsafeSlicePanic(ptr undef) #2
|
|
unreachable
|
|
}
|
|
|
|
declare void @runtime.unsafeSlicePanic(ptr) #0
|
|
|
|
; Function Attrs: nounwind
|
|
define hidden ptr @main.unsafeStringData(ptr %s.data, i32 %s.len, ptr %context) unnamed_addr #1 {
|
|
entry:
|
|
%stackalloc = alloca i8, align 1
|
|
call void @runtime.trackPointer(ptr %s.data, ptr nonnull %stackalloc, ptr undef) #2
|
|
ret ptr %s.data
|
|
}
|
|
|
|
attributes #0 = { "target-features"="+bulk-memory,+nontrapping-fptoint,+sign-ext" }
|
|
attributes #1 = { nounwind "target-features"="+bulk-memory,+nontrapping-fptoint,+sign-ext" }
|
|
attributes #2 = { nounwind }
|