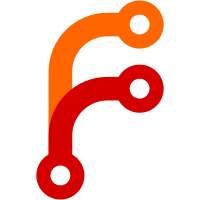
This PR adds a network device driver model called netdev. There will be a companion PR for TinyGo drivers to update the netdev drivers and network examples. This PR covers the core "net" package. An RFC for the work is here: #tinygo-org/drivers#487. Some things have changed from the RFC, but nothing major. The "net" package is a partial port of Go's "net" package, version 1.19.3. The src/net/README file has details on what is modified from Go's "net" package. Most "net" features are working as they would in normal Go. TCP/UDP/TLS protocol support is there. As well as HTTP client and server support. Standard Go network packages such as golang.org/x/net/websockets and Paho MQTT client work as-is. Other packages are likely to work as-is. Testing results are here (https://docs.google.com/spreadsheets/d/e/2PACX-1vT0cCjBvwXf9HJf6aJV2Sw198F2ief02gmbMV0sQocKT4y4RpfKv3dh6Jyew8lQW64FouZ8GwA2yjxI/pubhtml?gid=1013173032&single=true).
53 строки
1,6 КиБ
Go
53 строки
1,6 КиБ
Go
// Copyright 2017 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package syscall
|
|
|
|
// A RawConn is a raw network connection.
|
|
type RawConn interface {
|
|
// Control invokes f on the underlying connection's file
|
|
// descriptor or handle.
|
|
// The file descriptor fd is guaranteed to remain valid while
|
|
// f executes but not after f returns.
|
|
Control(f func(fd uintptr)) error
|
|
|
|
// Read invokes f on the underlying connection's file
|
|
// descriptor or handle; f is expected to try to read from the
|
|
// file descriptor.
|
|
// If f returns true, Read returns. Otherwise Read blocks
|
|
// waiting for the connection to be ready for reading and
|
|
// tries again repeatedly.
|
|
// The file descriptor is guaranteed to remain valid while f
|
|
// executes but not after f returns.
|
|
Read(f func(fd uintptr) (done bool)) error
|
|
|
|
// Write is like Read but for writing.
|
|
Write(f func(fd uintptr) (done bool)) error
|
|
}
|
|
|
|
// Conn is implemented by some types in the net and os packages to provide
|
|
// access to the underlying file descriptor or handle.
|
|
type Conn interface {
|
|
// SyscallConn returns a raw network connection.
|
|
SyscallConn() (RawConn, error)
|
|
}
|
|
|
|
const (
|
|
AF_INET = 0x2
|
|
SOCK_STREAM = 0x1
|
|
SOCK_DGRAM = 0x2
|
|
SOL_SOCKET = 0x1
|
|
SO_KEEPALIVE = 0x9
|
|
SOL_TCP = 0x6
|
|
TCP_KEEPINTVL = 0x5
|
|
IPPROTO_TCP = 0x6
|
|
IPPROTO_UDP = 0x11
|
|
F_SETFL = 0x4
|
|
|
|
// TINYGO: Made up, not a real IP protocol number. This is used to
|
|
// create a TLS socket on the device, assuming the device supports mbed
|
|
// TLS.
|
|
|
|
IPPROTO_TLS = 0xFE
|
|
)
|