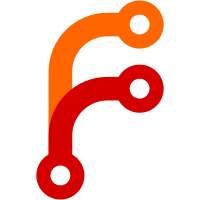
Let the standard library think that it is compiling for js/wasm. The most correct way of supporting bare metal Cortex-M targets would be using the 'arm' build tag and specifying no OS or an 'undefined' OS (perhaps GOOS=noos?). However, there is no build tag for specifying no OS at all, the closest possible is GOOS=js which makes very few assumptions. Sadly GOOS=js also makes some assumptions: it assumes to be running with GOARCH=wasm. This would not be such a problem, just add js, wasm and arm as build tags. However, having two GOARCH build tags leads to an error in internal/cpu: it defines variables for both architectures which then conflict. To work around these problems, the 'arm' target has been renamed to 'tinygo.arm', which should work around these problems. In the future, a GOOS=noos (or similar) should be added which can work with any architecture and doesn't implement OS-specific stuff.
28 строки
657 Б
Go
28 строки
657 Б
Go
// +build js,tinygo.arm avr
|
|
|
|
package runtime
|
|
|
|
// This file stubs out some external functions declared by the syscall/js
|
|
// package. They cannot be used on microcontrollers.
|
|
|
|
type js_ref uint64
|
|
|
|
//go:linkname js_valueGet syscall/js.valueGet
|
|
func js_valueGet(v js_ref, p string) js_ref {
|
|
return 0
|
|
}
|
|
|
|
//go:linkname js_valueNew syscall/js.valueNew
|
|
func js_valueNew(v js_ref, args []js_ref) (js_ref, bool) {
|
|
return 0, true
|
|
}
|
|
|
|
//go:linkname js_valueCall syscall/js.valueCall
|
|
func js_valueCall(v js_ref, m string, args []js_ref) (js_ref, bool) {
|
|
return 0, true
|
|
}
|
|
|
|
//go:linkname js_stringVal syscall/js.stringVal
|
|
func js_stringVal(x string) js_ref {
|
|
return 0
|
|
}
|