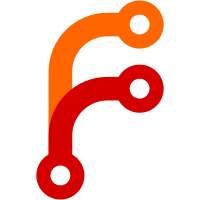
This allows better escape analysis even without being able to see the entire program. This makes the stack allocation test case more complete but probably won't have much of an effect outside of that (as the compiler is able to infer these attributes in the whole-program functionattrs pass).
58 строки
1,1 КиБ
Go
58 строки
1,1 КиБ
Go
package main
|
|
|
|
func main() {
|
|
n1 := 5
|
|
derefInt(&n1)
|
|
|
|
// This should eventually be modified to not escape.
|
|
n2 := 6 // OUT: object allocated on the heap: escapes at line 9
|
|
returnIntPtr(&n2)
|
|
|
|
s1 := make([]int, 3)
|
|
readIntSlice(s1)
|
|
|
|
s2 := [3]int{}
|
|
readIntSlice(s2[:])
|
|
|
|
// This should also be modified to not escape.
|
|
s3 := make([]int, 3) // OUT: object allocated on the heap: escapes at line 19
|
|
returnIntSlice(s3)
|
|
|
|
_ = make([]int, getUnknownNumber()) // OUT: object allocated on the heap: size is not constant
|
|
|
|
s4 := make([]byte, 300) // OUT: object allocated on the heap: object size 300 exceeds maximum stack allocation size 256
|
|
readByteSlice(s4)
|
|
|
|
s5 := make([]int, 4) // OUT: object allocated on the heap: escapes at line 27
|
|
s5 = append(s5, 5)
|
|
|
|
s6 := make([]int, 3)
|
|
s7 := []int{1, 2, 3}
|
|
copySlice(s6, s7)
|
|
}
|
|
|
|
func derefInt(x *int) int {
|
|
return *x
|
|
}
|
|
|
|
func returnIntPtr(x *int) *int {
|
|
return x
|
|
}
|
|
|
|
func readIntSlice(s []int) int {
|
|
return s[1]
|
|
}
|
|
|
|
func readByteSlice(s []byte) byte {
|
|
return s[1]
|
|
}
|
|
|
|
func returnIntSlice(s []int) []int {
|
|
return s
|
|
}
|
|
|
|
func getUnknownNumber() int
|
|
|
|
func copySlice(out, in []int) {
|
|
copy(out, in)
|
|
}
|