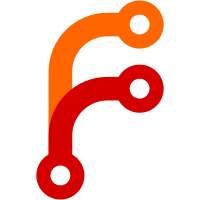
This can be used in the future to trigger garbage collection. For now, it provides a more useful error message in case the heap is completely filled up.
27 строки
516 Б
Go
27 строки
516 Б
Go
// +build wasm,!tinygo.arm,!avr
|
|
|
|
package runtime
|
|
|
|
import (
|
|
"unsafe"
|
|
)
|
|
|
|
const GOARCH = "wasm"
|
|
|
|
// The bitness of the CPU (e.g. 8, 32, 64).
|
|
const TargetBits = 32
|
|
|
|
//go:extern __heap_base
|
|
var heapStartSymbol unsafe.Pointer
|
|
|
|
var (
|
|
heapStart = uintptr(unsafe.Pointer(&heapStartSymbol))
|
|
heapEnd = (heapStart + wasmPageSize - 1) & (wasmPageSize - 1) // conservative guess: one page of heap memory
|
|
)
|
|
|
|
const wasmPageSize = 64 * 1024
|
|
|
|
// Align on word boundary.
|
|
func align(ptr uintptr) uintptr {
|
|
return (ptr + 3) &^ 3
|
|
}
|