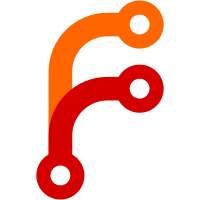
This can be very useful for some purposes: * It makes it possible to disable the UART in cases where it is not needed or needs to be disabled to conserve power. * It makes it possible to disable the serial output to reduce code size, which may be important for some chips. Sometimes, a few kB can be saved this way. * It makes it possible to override the default, for example you might want to use an actual UART to debug the USB-CDC implementation. It also lowers the dependency on having machine.Serial defined, which is often not defined when targeting a chip. Eventually, we might want to make it possible to write `-target=nrf52` or `-target=atmega328p` for example to target the chip itself with no board specific assumptions. The defaults don't change. I checked this by running `make smoketest` before and after and comparing the results.
85 строки
1,2 КиБ
Go
85 строки
1,2 КиБ
Go
// +build circuitplay_bluefruit
|
|
|
|
package machine
|
|
|
|
const HasLowFrequencyCrystal = false
|
|
|
|
// GPIO Pins
|
|
const (
|
|
D0 = P0_30
|
|
D1 = P0_14
|
|
D2 = P0_05
|
|
D3 = P0_04
|
|
D4 = P1_02
|
|
D5 = P1_15
|
|
D6 = P0_02
|
|
D7 = P1_06
|
|
D8 = P0_13
|
|
D9 = P0_29
|
|
D10 = P0_03
|
|
D11 = P1_04
|
|
D12 = P0_26
|
|
D13 = P1_14
|
|
)
|
|
|
|
// Analog Pins
|
|
const (
|
|
A1 = P0_02
|
|
A2 = P0_29
|
|
A3 = P0_03
|
|
A4 = P0_04
|
|
A5 = P0_05
|
|
A6 = P0_30
|
|
A7 = P0_14
|
|
A8 = P0_28
|
|
A9 = P0_31
|
|
)
|
|
|
|
const (
|
|
LED = D13
|
|
NEOPIXELS = D8
|
|
WS2812 = D8
|
|
|
|
BUTTONA = D4
|
|
BUTTONB = D5
|
|
SLIDER = D7 // built-in slide switch
|
|
|
|
BUTTON = BUTTONA
|
|
BUTTON1 = BUTTONB
|
|
|
|
LIGHTSENSOR = A8
|
|
TEMPSENSOR = A9
|
|
)
|
|
|
|
// UART0 pins (logical UART1)
|
|
const (
|
|
UART_TX_PIN = P0_14 // PORTB
|
|
UART_RX_PIN = P0_30 // PORTB
|
|
)
|
|
|
|
// I2C pins
|
|
const (
|
|
SDA_PIN = P0_05 // I2C0 external
|
|
SCL_PIN = P0_04 // I2C0 external
|
|
|
|
SDA1_PIN = P1_10 // I2C1 internal
|
|
SCL1_PIN = P1_12 // I2C1 internal
|
|
)
|
|
|
|
// SPI pins (internal flash)
|
|
const (
|
|
SPI0_SCK_PIN = P0_19 // SCK
|
|
SPI0_SDO_PIN = P0_21 // SDO
|
|
SPI0_SDI_PIN = P0_23 // SDI
|
|
)
|
|
|
|
// USB CDC identifiers
|
|
const (
|
|
usb_STRING_PRODUCT = "Adafruit Circuit Playground Bluefruit"
|
|
usb_STRING_MANUFACTURER = "Adafruit"
|
|
)
|
|
|
|
var (
|
|
usb_VID uint16 = 0x239A
|
|
usb_PID uint16 = 0x8045
|
|
)
|