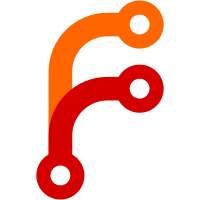
This can be useful to test improvements in LLVM master and to make it possible to support LLVM 11 for the most part already before the next release. That also allows catching LLVM bugs early to fix them upstream. Note that tests do not yet pass for this LLVM version, but the TinyGo compiler can be built with the binaries from apt.llvm.org (at the time of making this commit).
47 строки
1,6 КиБ
Go
47 строки
1,6 КиБ
Go
package transform
|
|
|
|
import "tinygo.org/x/go-llvm"
|
|
|
|
// This file implements small transformations on globals (functions and global
|
|
// variables) for specific ABIs/architectures.
|
|
|
|
// ApplyFunctionSections puts every function in a separate section. This makes
|
|
// it possible for the linker to remove dead code. It is the equivalent of
|
|
// passing -ffunction-sections to a C compiler.
|
|
func ApplyFunctionSections(mod llvm.Module) {
|
|
llvmFn := mod.FirstFunction()
|
|
for !llvmFn.IsNil() {
|
|
if !llvmFn.IsDeclaration() {
|
|
name := llvmFn.Name()
|
|
llvmFn.SetSection(".text." + name)
|
|
}
|
|
llvmFn = llvm.NextFunction(llvmFn)
|
|
}
|
|
}
|
|
|
|
// NonConstGlobals turns all global constants into global variables. This works
|
|
// around a limitation on Harvard architectures (e.g. AVR), where constant and
|
|
// non-constant pointers point to a different address space. Normal pointer
|
|
// behavior is restored by using the data space only, at the cost of RAM for
|
|
// constant global variables.
|
|
func NonConstGlobals(mod llvm.Module) {
|
|
global := mod.FirstGlobal()
|
|
for !global.IsNil() {
|
|
global.SetGlobalConstant(false)
|
|
global = llvm.NextGlobal(global)
|
|
}
|
|
}
|
|
|
|
// DisableTailCalls adds the "disable-tail-calls"="true" function attribute to
|
|
// all functions. This may be necessary, in particular to avoid an error with
|
|
// WebAssembly in LLVM 11.
|
|
func DisableTailCalls(mod llvm.Module) {
|
|
attribute := mod.Context().CreateStringAttribute("disable-tail-calls", "true")
|
|
llvmFn := mod.FirstFunction()
|
|
for !llvmFn.IsNil() {
|
|
if !llvmFn.IsDeclaration() {
|
|
llvmFn.AddFunctionAttr(attribute)
|
|
}
|
|
llvmFn = llvm.NextFunction(llvmFn)
|
|
}
|
|
}
|