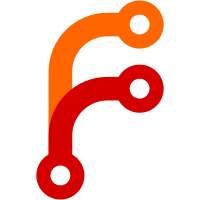
internal/itoa wasn't around back in go 1.12 days when tinygo's syscall/errno.go was written. It was only added as of go 1.17 ( https://github.com/golang/go/commit/061a6903a232cb868780b ) so we have to have an internal copy for now. The internal copy should be deleted when tinygo drops support for go 1.16. FWIW, the new version seems nicer. It uses no allocations when converting 0, and although the optimizer might make this moot, uses a multiplication x 10 instead of a mod operation.
32 строки
935 Б
Go
32 строки
935 Б
Go
package syscall
|
|
|
|
import "internal/itoa"
|
|
|
|
// Most code here has been copied from the Go sources:
|
|
// https://github.com/golang/go/blob/go1.12/src/syscall/syscall_js.go
|
|
// It has the following copyright note:
|
|
//
|
|
// Copyright 2018 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
// An Errno is an unsigned number describing an error condition.
|
|
// It implements the error interface. The zero Errno is by convention
|
|
// a non-error, so code to convert from Errno to error should use:
|
|
// err = nil
|
|
// if errno != 0 {
|
|
// err = errno
|
|
// }
|
|
type Errno uintptr
|
|
|
|
func (e Errno) Error() string {
|
|
return "errno " + itoa.Itoa(int(e))
|
|
}
|
|
|
|
func (e Errno) Temporary() bool {
|
|
return e == EINTR || e == EMFILE || e.Timeout()
|
|
}
|
|
|
|
func (e Errno) Timeout() bool {
|
|
return e == EAGAIN || e == EWOULDBLOCK || e == ETIMEDOUT
|
|
}
|