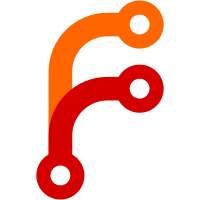
This gives some more optimization opportunities to LLVM, because it understands these intrinsics. For example, it might convert llvm.sqrt.f64 to llvm.sqrt.f32 if possible.
36 строки
1,9 КиБ
LLVM
36 строки
1,9 КиБ
LLVM
; ModuleID = 'intrinsics.go'
|
|
source_filename = "intrinsics.go"
|
|
target datalayout = "e-m:e-p:32:32-Fi8-i64:64-v128:64:128-a:0:32-n32-S64"
|
|
target triple = "thumbv7m-unknown-unknown-eabi"
|
|
|
|
declare noalias nonnull i8* @runtime.alloc(i32, i8*, i8*) #0
|
|
|
|
; Function Attrs: nounwind
|
|
define hidden void @main.init(i8* %context) unnamed_addr #1 {
|
|
entry:
|
|
ret void
|
|
}
|
|
|
|
; Function Attrs: nounwind
|
|
define hidden double @main.mySqrt(double %x, i8* %context) unnamed_addr #1 {
|
|
entry:
|
|
%0 = call double @llvm.sqrt.f64(double %x)
|
|
ret double %0
|
|
}
|
|
|
|
; Function Attrs: nofree nosync nounwind readnone speculatable willreturn
|
|
declare double @llvm.sqrt.f64(double) #2
|
|
|
|
; Function Attrs: nounwind
|
|
define hidden double @main.myTrunc(double %x, i8* %context) unnamed_addr #1 {
|
|
entry:
|
|
%0 = call double @llvm.trunc.f64(double %x)
|
|
ret double %0
|
|
}
|
|
|
|
; Function Attrs: nofree nosync nounwind readnone speculatable willreturn
|
|
declare double @llvm.trunc.f64(double) #2
|
|
|
|
attributes #0 = { "target-features"="+armv7-m,+hwdiv,+soft-float,+strict-align,+thumb-mode,-aes,-bf16,-cdecp0,-cdecp1,-cdecp2,-cdecp3,-cdecp4,-cdecp5,-cdecp6,-cdecp7,-crc,-crypto,-d32,-dotprod,-dsp,-fp-armv8,-fp-armv8d16,-fp-armv8d16sp,-fp-armv8sp,-fp16,-fp16fml,-fp64,-fpregs,-fullfp16,-hwdiv-arm,-i8mm,-lob,-mve,-mve.fp,-neon,-pacbti,-ras,-sb,-sha2,-vfp2,-vfp2sp,-vfp3,-vfp3d16,-vfp3d16sp,-vfp3sp,-vfp4,-vfp4d16,-vfp4d16sp,-vfp4sp" }
|
|
attributes #1 = { nounwind "target-features"="+armv7-m,+hwdiv,+soft-float,+strict-align,+thumb-mode,-aes,-bf16,-cdecp0,-cdecp1,-cdecp2,-cdecp3,-cdecp4,-cdecp5,-cdecp6,-cdecp7,-crc,-crypto,-d32,-dotprod,-dsp,-fp-armv8,-fp-armv8d16,-fp-armv8d16sp,-fp-armv8sp,-fp16,-fp16fml,-fp64,-fpregs,-fullfp16,-hwdiv-arm,-i8mm,-lob,-mve,-mve.fp,-neon,-pacbti,-ras,-sb,-sha2,-vfp2,-vfp2sp,-vfp3,-vfp3d16,-vfp3d16sp,-vfp3sp,-vfp4,-vfp4d16,-vfp4d16sp,-vfp4sp" }
|
|
attributes #2 = { nofree nosync nounwind readnone speculatable willreturn }
|