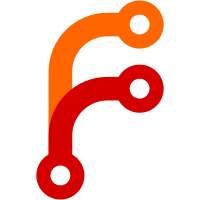
On Debian, all LLVM commands have a version suffix (clang-8, ld.lld-8, wasm-ld-8, etc.). However. Most other distributions only provide a version prefix for Clang and not for all the other commands. This commit fixes the issue by trying the command with the version suffix first and falling back to one without if needed.
33 строки
810 Б
Go
33 строки
810 Б
Go
package main
|
|
|
|
import (
|
|
"errors"
|
|
"os"
|
|
"os/exec"
|
|
"strings"
|
|
)
|
|
|
|
// Commands used by the compilation process might have different file names
|
|
// across operating systems and distributions.
|
|
var commands = map[string][]string{
|
|
"clang": {"clang-8"},
|
|
"ld.lld": {"ld.lld-8", "ld.lld"},
|
|
"wasm-ld": {"wasm-ld-8", "wasm-ld"},
|
|
}
|
|
|
|
func execCommand(cmdNames []string, args ...string) error {
|
|
for _, cmdName := range cmdNames {
|
|
cmd := exec.Command(cmdName, args...)
|
|
cmd.Stdout = os.Stdout
|
|
cmd.Stderr = os.Stderr
|
|
err := cmd.Run()
|
|
if err != nil {
|
|
if err, ok := err.(*exec.Error); ok && err.Err == exec.ErrNotFound {
|
|
// this command was not found, try the next
|
|
continue
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
return errors.New("none of these commands were found in your $PATH: " + strings.Join(cmdNames, " "))
|
|
}
|