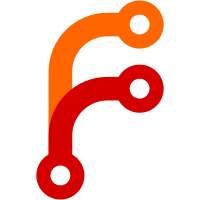
For a full explanation, see interp/README.md. In short, this rewrite is a redesign of the partial evaluator which improves it over the previous partial evaluator. The main functional difference is that when interpreting a function, the interpretation can be rolled back when an unsupported instruction is encountered (for example, an actual unknown instruction or a branch on a value that's only known at runtime). This also means that it is no longer necessary to scan functions to see whether they can be interpreted: instead, this package now just tries to interpret it and reverts when it can't go further. This new design has several benefits: * Most errors coming from the interp package are avoided, as it can simply skip the code it can't handle. This has long been an issue. * The memory model has been improved, which means some packages now pass all tests that previously didn't pass them. * Because of a better design, it is in fact a bit faster than the previous version. This means the following packages now pass tests with `tinygo test`: * hash/adler32: previously it would hang in an infinite loop * math/cmplx: previously it resulted in errors This also means that the math/big package can be imported. It would previously fail with a "interp: branch on a non-constant" error.
46 строки
1,6 КиБ
LLVM
46 строки
1,6 КиБ
LLVM
target datalayout = "e-m:e-i64:64-f80:128-n8:16:32:64-S128"
|
|
target triple = "x86_64--linux"
|
|
|
|
@main.nonConst1 = local_unnamed_addr global [4 x i64] zeroinitializer
|
|
@main.nonConst2 = local_unnamed_addr global i64 0
|
|
@main.someArray = global [8 x { i16, i32 }] zeroinitializer
|
|
@main.exportedValue = global [1 x i16*] [i16* @main.exposedValue1]
|
|
@main.exposedValue1 = global i16 0
|
|
@main.exposedValue2 = local_unnamed_addr global i16 0
|
|
|
|
declare void @runtime.printint64(i64) unnamed_addr
|
|
|
|
declare void @runtime.printnl() unnamed_addr
|
|
|
|
define void @runtime.initAll() unnamed_addr {
|
|
entry:
|
|
call void @runtime.printint64(i64 5)
|
|
call void @runtime.printnl()
|
|
%value1 = call i64 @someValue()
|
|
store i64 %value1, i64* getelementptr inbounds ([4 x i64], [4 x i64]* @main.nonConst1, i32 0, i32 0)
|
|
%value2 = load i64, i64* getelementptr inbounds ([4 x i64], [4 x i64]* @main.nonConst1, i32 0, i32 0)
|
|
store i64 %value2, i64* @main.nonConst2
|
|
call void @modifyExternal(i32* getelementptr inbounds ([8 x { i16, i32 }], [8 x { i16, i32 }]* @main.someArray, i32 0, i32 3, i32 1))
|
|
call void @modifyExternal(i32* bitcast ([1 x i16*]* @main.exportedValue to i32*))
|
|
store i16 5, i16* @main.exposedValue1
|
|
call void @modifyExternal(i32* bitcast (void ()* @willModifyGlobal to i32*))
|
|
store i16 7, i16* @main.exposedValue2
|
|
ret void
|
|
}
|
|
|
|
define void @main() unnamed_addr {
|
|
entry:
|
|
call void @runtime.printint64(i64 3)
|
|
call void @runtime.printnl()
|
|
ret void
|
|
}
|
|
|
|
declare i64 @someValue() local_unnamed_addr
|
|
|
|
declare void @modifyExternal(i32*) local_unnamed_addr
|
|
|
|
define void @willModifyGlobal() {
|
|
entry:
|
|
store i16 8, i16* @main.exposedValue2
|
|
ret void
|
|
}
|