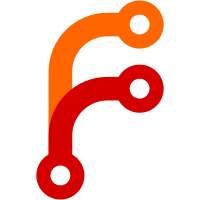
These two passes are related, but can definitely work independently. Which is what this change does: it splits the two passes. This should make it easier to change these two new passes in the future. This change now also enables slightly better testing by testing these two passes independently. In particular, the reflect lowering pass got some actual tests: it was barely unit-tested before. I have verified that this doesn't really change code size, at least not on the microbit target. Two tests do change, but in a very minor way (and in opposite direction).
80 строки
2 КиБ
Go
80 строки
2 КиБ
Go
package transform_test
|
|
|
|
import (
|
|
"go/token"
|
|
"io/ioutil"
|
|
"path/filepath"
|
|
"regexp"
|
|
"sort"
|
|
"strconv"
|
|
"strings"
|
|
"testing"
|
|
|
|
"github.com/tinygo-org/tinygo/transform"
|
|
"tinygo.org/x/go-llvm"
|
|
)
|
|
|
|
func TestAllocs(t *testing.T) {
|
|
t.Parallel()
|
|
testTransform(t, "testdata/allocs", func(mod llvm.Module) {
|
|
transform.OptimizeAllocs(mod, nil, nil)
|
|
})
|
|
}
|
|
|
|
type allocsTestOutput struct {
|
|
filename string
|
|
line int
|
|
msg string
|
|
}
|
|
|
|
func (out allocsTestOutput) String() string {
|
|
return out.filename + ":" + strconv.Itoa(out.line) + ": " + out.msg
|
|
}
|
|
|
|
// Test with a Go file as input (for more accurate tests).
|
|
func TestAllocs2(t *testing.T) {
|
|
t.Parallel()
|
|
|
|
mod := compileGoFileForTesting(t, "./testdata/allocs2.go")
|
|
|
|
// Run functionattrs pass, which is necessary for escape analysis.
|
|
pm := llvm.NewPassManager()
|
|
defer pm.Dispose()
|
|
pm.AddInstructionCombiningPass()
|
|
pm.AddFunctionAttrsPass()
|
|
pm.Run(mod)
|
|
|
|
// Run heap to stack transform.
|
|
var testOutputs []allocsTestOutput
|
|
transform.OptimizeAllocs(mod, regexp.MustCompile("."), func(pos token.Position, msg string) {
|
|
testOutputs = append(testOutputs, allocsTestOutput{
|
|
filename: filepath.Base(pos.Filename),
|
|
line: pos.Line,
|
|
msg: msg,
|
|
})
|
|
})
|
|
sort.Slice(testOutputs, func(i, j int) bool {
|
|
return testOutputs[i].line < testOutputs[j].line
|
|
})
|
|
testOutput := ""
|
|
for _, out := range testOutputs {
|
|
testOutput += out.String() + "\n"
|
|
}
|
|
|
|
// Load expected test output (the OUT: lines).
|
|
testInput, err := ioutil.ReadFile("./testdata/allocs2.go")
|
|
if err != nil {
|
|
t.Fatal("could not read test input:", err)
|
|
}
|
|
var expectedTestOutput string
|
|
for i, line := range strings.Split(strings.ReplaceAll(string(testInput), "\r\n", "\n"), "\n") {
|
|
if idx := strings.Index(line, " // OUT: "); idx > 0 {
|
|
msg := line[idx+len(" // OUT: "):]
|
|
expectedTestOutput += "allocs2.go:" + strconv.Itoa(i+1) + ": " + msg + "\n"
|
|
}
|
|
}
|
|
|
|
if testOutput != expectedTestOutput {
|
|
t.Errorf("output does not match expected output:\n%s", testOutput)
|
|
}
|
|
}
|