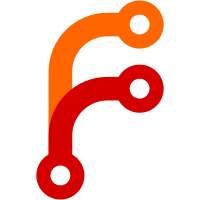
This change adds support for the ESP32-C3, a new chip from Espressif. It is a RISC-V core so porting was comparatively easy. Most peripherals are shared with the (original) ESP32 chip, but with subtle differences. Also, the SVD file I've used gives some peripherals/registers a different name which makes sharing code harder. Eventually, when an official SVD file for the ESP32 is released, I expect that a lot of code can be shared between the two chips. More information: https://www.espressif.com/en/products/socs/esp32-c3 TODO: - stack scheduler - interrupts - most peripherals (SPI, I2C, PWM, etc)
69 строки
1,9 КиБ
Go
69 строки
1,9 КиБ
Go
// +build esp32 esp32c3
|
|
|
|
package runtime
|
|
|
|
import (
|
|
"device/esp"
|
|
"machine"
|
|
"unsafe"
|
|
)
|
|
|
|
type timeUnit int64
|
|
|
|
func putchar(c byte) {
|
|
machine.Serial.WriteByte(c)
|
|
}
|
|
|
|
func postinit() {}
|
|
|
|
// Initialize .bss: zero-initialized global variables.
|
|
// The .data section has already been loaded by the ROM bootloader.
|
|
func clearbss() {
|
|
ptr := unsafe.Pointer(&_sbss)
|
|
for ptr != unsafe.Pointer(&_ebss) {
|
|
*(*uint32)(ptr) = 0
|
|
ptr = unsafe.Pointer(uintptr(ptr) + 4)
|
|
}
|
|
}
|
|
|
|
func initTimer() {
|
|
// Configure timer 0 in timer group 0, for timekeeping.
|
|
// EN: Enable the timer.
|
|
// INCREASE: Count up every tick (as opposed to counting down).
|
|
// DIVIDER: 16-bit prescaler, set to 2 for dividing the APB clock by two
|
|
// (40MHz).
|
|
esp.TIMG0.T0CONFIG.Set(esp.TIMG_T0CONFIG_T0_EN | esp.TIMG_T0CONFIG_T0_INCREASE | 2<<esp.TIMG_T0CONFIG_T0_DIVIDER_Pos)
|
|
|
|
// Set the timer counter value to 0.
|
|
esp.TIMG0.T0LOADLO.Set(0)
|
|
esp.TIMG0.T0LOADHI.Set(0)
|
|
esp.TIMG0.T0LOAD.Set(0) // value doesn't matter.
|
|
}
|
|
|
|
func ticks() timeUnit {
|
|
// First, update the LO and HI register pair by writing any value to the
|
|
// register. This allows reading the pair atomically.
|
|
esp.TIMG0.T0UPDATE.Set(0)
|
|
// Then read the two 32-bit parts of the timer.
|
|
return timeUnit(uint64(esp.TIMG0.T0LO.Get()) | uint64(esp.TIMG0.T0HI.Get())<<32)
|
|
}
|
|
|
|
func nanosecondsToTicks(ns int64) timeUnit {
|
|
// Calculate the number of ticks from the number of nanoseconds. At a 80MHz
|
|
// APB clock, that's 25 nanoseconds per tick with a timer prescaler of 2:
|
|
// 25 = 1e9 / (80MHz / 2)
|
|
return timeUnit(ns / 25)
|
|
}
|
|
|
|
func ticksToNanoseconds(ticks timeUnit) int64 {
|
|
// See nanosecondsToTicks.
|
|
return int64(ticks) * 25
|
|
}
|
|
|
|
// sleepTicks busy-waits until the given number of ticks have passed.
|
|
func sleepTicks(d timeUnit) {
|
|
sleepUntil := ticks() + d
|
|
for ticks() < sleepUntil {
|
|
// TODO: suspend the CPU to not burn power here unnecessarily.
|
|
}
|
|
}
|