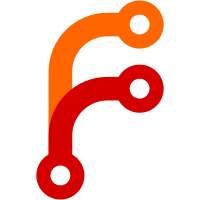
The division and remainder operations were lowered directly to LLVM IR. This is wrong however because the Go specification defines exactly what happens on a divide by zero or signed integer overflow and LLVM IR itself treats those cases as undefined behavior. Therefore, this commit implements divide by zero and signed integer overflow according to the Go specification. This does have an impact on the generated code, but it is surprisingly small. I've used the drivers repo to test the code before and after, and to my surprise most driver smoke tests are not changed at all. Those that are, have only a small increase in code size. At the same time, this change makes TinyGo more compliant to the Go specification.
88 строки
1,3 КиБ
Go
88 строки
1,3 КиБ
Go
package main
|
|
|
|
// Basic tests that don't need to be split into a separate file.
|
|
|
|
func addInt(x, y int) int {
|
|
return x + y
|
|
}
|
|
|
|
func equalInt(x, y int) bool {
|
|
return x == y
|
|
}
|
|
|
|
func divInt(x, y int) int {
|
|
return x / y
|
|
}
|
|
|
|
func divUint(x, y uint) uint {
|
|
return x / y
|
|
}
|
|
|
|
func remInt(x, y int) int {
|
|
return x % y
|
|
}
|
|
|
|
func remUint(x, y uint) uint {
|
|
return x % y
|
|
}
|
|
|
|
func floatEQ(x, y float32) bool {
|
|
return x == y
|
|
}
|
|
|
|
func floatNE(x, y float32) bool {
|
|
return x != y
|
|
}
|
|
|
|
func floatLower(x, y float32) bool {
|
|
return x < y
|
|
}
|
|
|
|
func floatLowerEqual(x, y float32) bool {
|
|
return x <= y
|
|
}
|
|
|
|
func floatGreater(x, y float32) bool {
|
|
return x > y
|
|
}
|
|
|
|
func floatGreaterEqual(x, y float32) bool {
|
|
return x >= y
|
|
}
|
|
|
|
func complexReal(x complex64) float32 {
|
|
return real(x)
|
|
}
|
|
|
|
func complexImag(x complex64) float32 {
|
|
return imag(x)
|
|
}
|
|
|
|
func complexAdd(x, y complex64) complex64 {
|
|
return x + y
|
|
}
|
|
|
|
func complexSub(x, y complex64) complex64 {
|
|
return x - y
|
|
}
|
|
|
|
func complexMul(x, y complex64) complex64 {
|
|
return x * y
|
|
}
|
|
|
|
// TODO: complexDiv (requires runtime call)
|
|
|
|
// A type 'kv' also exists in function foo. Test that these two types don't
|
|
// conflict with each other.
|
|
type kv struct {
|
|
v float32
|
|
}
|
|
|
|
func foo(a *kv) {
|
|
// Define a new 'kv' type.
|
|
type kv struct {
|
|
v byte
|
|
}
|
|
// Use this type.
|
|
func(b *kv) {}(nil)
|
|
}
|