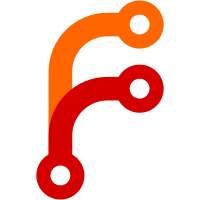
This function previously returned the atomic time, that isn't affected by system time changes but also has a time base at some arbitrary time in the past. This makes sense for baremetal platforms (which typically don't know the wall time) but it gives surprising results on Linux and macOS: time.Now() usually returns a time somewhere near the start of 1970. This commit fixes this by obtaining both time values: the monotonic time and the wall clock time. This is also how the Go runtime implements the time.now function.
75 строки
1,7 КиБ
Go
75 строки
1,7 КиБ
Go
// +build baremetal
|
|
|
|
package runtime
|
|
|
|
import (
|
|
"unsafe"
|
|
)
|
|
|
|
//go:extern _heap_start
|
|
var heapStartSymbol [0]byte
|
|
|
|
//go:extern _heap_end
|
|
var heapEndSymbol [0]byte
|
|
|
|
//go:extern _globals_start
|
|
var globalsStartSymbol [0]byte
|
|
|
|
//go:extern _globals_end
|
|
var globalsEndSymbol [0]byte
|
|
|
|
//go:extern _stack_top
|
|
var stackTopSymbol [0]byte
|
|
|
|
var (
|
|
heapStart = uintptr(unsafe.Pointer(&heapStartSymbol))
|
|
heapEnd = uintptr(unsafe.Pointer(&heapEndSymbol))
|
|
globalsStart = uintptr(unsafe.Pointer(&globalsStartSymbol))
|
|
globalsEnd = uintptr(unsafe.Pointer(&globalsEndSymbol))
|
|
stackTop = uintptr(unsafe.Pointer(&stackTopSymbol))
|
|
)
|
|
|
|
// growHeap tries to grow the heap size. It returns true if it succeeds, false
|
|
// otherwise.
|
|
func growHeap() bool {
|
|
// On baremetal, there is no way the heap can be grown.
|
|
return false
|
|
}
|
|
|
|
//export malloc
|
|
func libc_malloc(size uintptr) unsafe.Pointer {
|
|
return alloc(size)
|
|
}
|
|
|
|
//export free
|
|
func libc_free(ptr unsafe.Pointer) {
|
|
free(ptr)
|
|
}
|
|
|
|
//go:linkname syscall_Exit syscall.Exit
|
|
func syscall_Exit(code int) {
|
|
abort()
|
|
}
|
|
|
|
const baremetal = true
|
|
|
|
// timeOffset is how long the monotonic clock started after the Unix epoch. It
|
|
// should be a positive integer under normal operation or zero when it has not
|
|
// been set.
|
|
var timeOffset int64
|
|
|
|
//go:linkname now time.now
|
|
func now() (sec int64, nsec int32, mono int64) {
|
|
mono = nanotime()
|
|
sec = (mono + timeOffset) / (1000 * 1000 * 1000)
|
|
nsec = int32((mono + timeOffset) - sec*(1000*1000*1000))
|
|
return
|
|
}
|
|
|
|
// AdjustTimeOffset adds the given offset to the built-in time offset. A
|
|
// positive value adds to the time (skipping some time), a negative value moves
|
|
// the clock into the past.
|
|
func AdjustTimeOffset(offset int64) {
|
|
// TODO: do this atomically?
|
|
timeOffset += offset
|
|
}
|