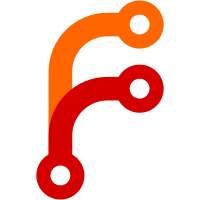
Previously, the machine.UART0 object had two meanings: - it was the first UART on the chip - it was the default output for println These two meanings conflict, and resulted in workarounds like: - Defining UART0 to refer to the USB-CDC interface (atsamd21, atsamd51, nrf52840), even though that clearly isn't an UART. - Defining NRF_UART0 to avoid a conflict with UART0 (which was redefined as a USB-CDC interface). - Defining aliases like UART0 = UART1, which refer to the same hardware peripheral (stm32). This commit changes this to use a new machine.Serial object for the default serial port. It might refer to the first or second UART depending on the board, or even to the USB-CDC interface. Also, UART0 now really refers to the first UART on the chip, no longer to a USB-CDC interface. The changes in the runtime package are all just search+replace. The changes in the machine package are a mixture of search+replace and manual modifications. This commit does not affect binary size, in fact it doesn't affect the resulting binary at all.
44 строки
1,1 КиБ
Go
44 строки
1,1 КиБ
Go
// +build avr,atmega
|
|
|
|
package runtime
|
|
|
|
import (
|
|
"device/avr"
|
|
"machine"
|
|
)
|
|
|
|
func initUART() {
|
|
machine.Serial.Configure(machine.UARTConfig{})
|
|
}
|
|
|
|
func putchar(c byte) {
|
|
machine.Serial.WriteByte(c)
|
|
}
|
|
|
|
// Sleep for a given period. The period is defined by the WDT peripheral, and is
|
|
// on most chips (at least) 3 bits wide, in powers of two from 16ms to 2s
|
|
// (0=16ms, 1=32ms, 2=64ms...). Note that the WDT is not very accurate: it can
|
|
// be off by a large margin depending on temperature and supply voltage.
|
|
//
|
|
// TODO: disable more peripherals etc. to reduce sleep current.
|
|
func sleepWDT(period uint8) {
|
|
// Configure WDT
|
|
avr.Asm("cli")
|
|
avr.Asm("wdr")
|
|
// Start timed sequence.
|
|
avr.WDTCSR.SetBits(avr.WDTCSR_WDCE | avr.WDTCSR_WDE)
|
|
// Enable WDT and set new timeout
|
|
avr.WDTCSR.SetBits(avr.WDTCSR_WDIE | period)
|
|
avr.Asm("sei")
|
|
|
|
// Set sleep mode to idle and enable sleep mode.
|
|
// Note: when using something other than idle, the UART won't work
|
|
// correctly. This needs to be fixed, though, so we can truly sleep.
|
|
avr.SMCR.Set((0 << 1) | avr.SMCR_SE)
|
|
|
|
// go to sleep
|
|
avr.Asm("sleep")
|
|
|
|
// disable sleep
|
|
avr.SMCR.Set(0)
|
|
}
|