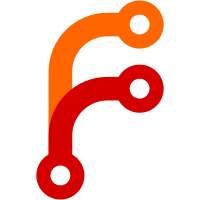
Previously, the machine.UART0 object had two meanings: - it was the first UART on the chip - it was the default output for println These two meanings conflict, and resulted in workarounds like: - Defining UART0 to refer to the USB-CDC interface (atsamd21, atsamd51, nrf52840), even though that clearly isn't an UART. - Defining NRF_UART0 to avoid a conflict with UART0 (which was redefined as a USB-CDC interface). - Defining aliases like UART0 = UART1, which refer to the same hardware peripheral (stm32). This commit changes this to use a new machine.Serial object for the default serial port. It might refer to the first or second UART depending on the board, or even to the USB-CDC interface. Also, UART0 now really refers to the first UART on the chip, no longer to a USB-CDC interface. The changes in the runtime package are all just search+replace. The changes in the machine package are a mixture of search+replace and manual modifications. This commit does not affect binary size, in fact it doesn't affect the resulting binary at all.
167 строки
3,5 КиБ
Go
167 строки
3,5 КиБ
Go
// +build !baremetal
|
|
|
|
package machine
|
|
|
|
// Dummy machine package that calls out to external functions.
|
|
|
|
var (
|
|
SPI0 = SPI{0}
|
|
I2C0 = &I2C{0}
|
|
UART0 = &UART{0}
|
|
USB = &UART{100}
|
|
)
|
|
|
|
const (
|
|
PinInput PinMode = iota
|
|
PinOutput
|
|
PinInputPullup
|
|
PinInputPulldown
|
|
)
|
|
|
|
func (p Pin) Configure(config PinConfig) {
|
|
gpioConfigure(p, config)
|
|
}
|
|
|
|
func (p Pin) Set(value bool) {
|
|
gpioSet(p, value)
|
|
}
|
|
|
|
func (p Pin) Get() bool {
|
|
return gpioGet(p)
|
|
}
|
|
|
|
//export __tinygo_gpio_configure
|
|
func gpioConfigure(pin Pin, config PinConfig)
|
|
|
|
//export __tinygo_gpio_set
|
|
func gpioSet(pin Pin, value bool)
|
|
|
|
//export __tinygo_gpio_get
|
|
func gpioGet(pin Pin) bool
|
|
|
|
type SPI struct {
|
|
Bus uint8
|
|
}
|
|
|
|
type SPIConfig struct {
|
|
Frequency uint32
|
|
SCK Pin
|
|
SDO Pin
|
|
SDI Pin
|
|
Mode uint8
|
|
}
|
|
|
|
func (spi SPI) Configure(config SPIConfig) {
|
|
spiConfigure(spi.Bus, config.SCK, config.SDO, config.SDI)
|
|
}
|
|
|
|
// Transfer writes/reads a single byte using the SPI interface.
|
|
func (spi SPI) Transfer(w byte) (byte, error) {
|
|
return spiTransfer(spi.Bus, w), nil
|
|
}
|
|
|
|
//export __tinygo_spi_configure
|
|
func spiConfigure(bus uint8, sck Pin, SDO Pin, SDI Pin)
|
|
|
|
//export __tinygo_spi_transfer
|
|
func spiTransfer(bus uint8, w uint8) uint8
|
|
|
|
// InitADC enables support for ADC peripherals.
|
|
func InitADC() {
|
|
// Nothing to do here.
|
|
}
|
|
|
|
// Configure configures an ADC pin to be able to be used to read data.
|
|
func (adc ADC) Configure(ADCConfig) {
|
|
}
|
|
|
|
// Get reads the current analog value from this ADC peripheral.
|
|
func (adc ADC) Get() uint16 {
|
|
return adcRead(adc.Pin)
|
|
}
|
|
|
|
//export __tinygo_adc_read
|
|
func adcRead(pin Pin) uint16
|
|
|
|
// I2C is a generic implementation of the Inter-IC communication protocol.
|
|
type I2C struct {
|
|
Bus uint8
|
|
}
|
|
|
|
// I2CConfig is used to store config info for I2C.
|
|
type I2CConfig struct {
|
|
Frequency uint32
|
|
SCL Pin
|
|
SDA Pin
|
|
}
|
|
|
|
// Configure is intended to setup the I2C interface.
|
|
func (i2c *I2C) Configure(config I2CConfig) error {
|
|
i2cConfigure(i2c.Bus, config.SCL, config.SDA)
|
|
return nil
|
|
}
|
|
|
|
// Tx does a single I2C transaction at the specified address.
|
|
func (i2c *I2C) Tx(addr uint16, w, r []byte) error {
|
|
i2cTransfer(i2c.Bus, &w[0], len(w), &r[0], len(r))
|
|
// TODO: do something with the returned error code.
|
|
return nil
|
|
}
|
|
|
|
//export __tinygo_i2c_configure
|
|
func i2cConfigure(bus uint8, scl Pin, sda Pin)
|
|
|
|
//export __tinygo_i2c_transfer
|
|
func i2cTransfer(bus uint8, w *byte, wlen int, r *byte, rlen int) int
|
|
|
|
type UART struct {
|
|
Bus uint8
|
|
}
|
|
|
|
type UARTConfig struct {
|
|
BaudRate uint32
|
|
TX Pin
|
|
RX Pin
|
|
}
|
|
|
|
// Configure the UART.
|
|
func (uart *UART) Configure(config UARTConfig) {
|
|
uartConfigure(uart.Bus, config.TX, config.RX)
|
|
}
|
|
|
|
// Read from the UART.
|
|
func (uart *UART) Read(data []byte) (n int, err error) {
|
|
return uartRead(uart.Bus, &data[0], len(data)), nil
|
|
}
|
|
|
|
// Write to the UART.
|
|
func (uart *UART) Write(data []byte) (n int, err error) {
|
|
return uartWrite(uart.Bus, &data[0], len(data)), nil
|
|
}
|
|
|
|
// Buffered returns the number of bytes currently stored in the RX buffer.
|
|
func (uart *UART) Buffered() int {
|
|
return 0
|
|
}
|
|
|
|
// ReadByte reads a single byte from the UART.
|
|
func (uart *UART) ReadByte() (byte, error) {
|
|
var b byte
|
|
uartRead(uart.Bus, &b, 1)
|
|
return b, nil
|
|
}
|
|
|
|
// WriteByte writes a single byte to the UART.
|
|
func (uart *UART) WriteByte(b byte) error {
|
|
uartWrite(uart.Bus, &b, 1)
|
|
return nil
|
|
}
|
|
|
|
//export __tinygo_uart_configure
|
|
func uartConfigure(bus uint8, tx Pin, rx Pin)
|
|
|
|
//export __tinygo_uart_read
|
|
func uartRead(bus uint8, buf *byte, bufLen int) int
|
|
|
|
//export __tinygo_uart_write
|
|
func uartWrite(bus uint8, buf *byte, bufLen int) int
|