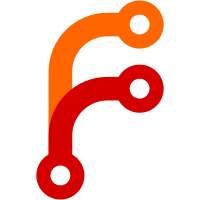
I have checked this conversion is not needed anymore after the previous commit, by running various smoke tests of which none triggered this optimization. The only case where the optimization would have kicked in is in syscall/syscall_windows.go:76 of the Go standard library. Therefore, I prefer to remove it to reduce code complexity.
26 строки
558 Б
Go
26 строки
558 Б
Go
package main
|
|
|
|
// This file tests various operations on pointers, such as pointer arithmetic
|
|
// and dereferencing pointers.
|
|
|
|
import "unsafe"
|
|
|
|
// Dereference pointers.
|
|
|
|
func pointerDerefZero(x *[0]int) [0]int {
|
|
return *x // This is a no-op, there is nothing to load.
|
|
}
|
|
|
|
// Unsafe pointer casts, they are sometimes a no-op.
|
|
|
|
func pointerCastFromUnsafe(x unsafe.Pointer) *int {
|
|
return (*int)(x)
|
|
}
|
|
|
|
func pointerCastToUnsafe(x *int) unsafe.Pointer {
|
|
return unsafe.Pointer(x)
|
|
}
|
|
|
|
func pointerCastToUnsafeNoop(x *byte) unsafe.Pointer {
|
|
return unsafe.Pointer(x)
|
|
}
|