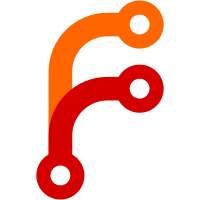
Unfortunately the calling convention for variadic functions is different from the calling convention of regular functions on darwin/arm64, and open happens to be such a variadic function. The syscall package treated it like a regular function, which resulted in buggy behavior. This fix introduces a wrapper function. This is the cleanest change I could come up with.
78 строки
1,1 КиБ
Go
78 строки
1,1 КиБ
Go
//go:build nintendoswitch
|
|
// +build nintendoswitch
|
|
|
|
package syscall
|
|
|
|
import (
|
|
"internal/itoa"
|
|
)
|
|
|
|
// A Signal is a number describing a process signal.
|
|
// It implements the os.Signal interface.
|
|
type Signal int
|
|
|
|
const (
|
|
_ Signal = iota
|
|
SIGCHLD
|
|
SIGINT
|
|
SIGKILL
|
|
SIGTRAP
|
|
SIGQUIT
|
|
SIGTERM
|
|
)
|
|
|
|
func (s Signal) Signal() {}
|
|
|
|
func (s Signal) String() string {
|
|
if 0 <= s && int(s) < len(signals) {
|
|
str := signals[s]
|
|
if str != "" {
|
|
return str
|
|
}
|
|
}
|
|
return "signal " + itoa.Itoa(int(s))
|
|
}
|
|
|
|
var signals = [...]string{}
|
|
|
|
// File system
|
|
|
|
const (
|
|
Stdin = 0
|
|
Stdout = 1
|
|
Stderr = 2
|
|
)
|
|
|
|
const (
|
|
O_RDONLY = 0
|
|
O_WRONLY = 1
|
|
O_RDWR = 2
|
|
|
|
O_CREAT = 0100
|
|
O_TRUNC = 01000
|
|
O_APPEND = 02000
|
|
O_EXCL = 0200
|
|
O_SYNC = 010000
|
|
|
|
O_CLOEXEC = 0
|
|
)
|
|
|
|
//go:extern errno
|
|
var libcErrno uintptr
|
|
|
|
func getErrno() error {
|
|
return Errno(libcErrno)
|
|
}
|
|
|
|
func Pipe2(p []int, flags int) (err error) {
|
|
return ENOSYS // TODO
|
|
}
|
|
|
|
func Getpagesize() int {
|
|
return 4096 // TODO
|
|
}
|
|
|
|
// int open(const char *pathname, int flags, mode_t mode);
|
|
//
|
|
//export open
|
|
func libc_open(pathname *byte, flags int32, mode uint32) int32
|