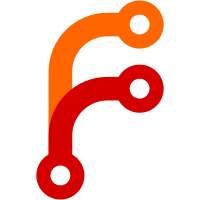
There were a few issues that were causing qemu-system-arm and qemu-system-riscv to give the wrong exit codes. They are in fact capable of exiting with 0 or 1 signalled from the running application, but this functionality wasn't used. This commit changes this in the following ways: * It fixes SemiHosting codes, which were incorrectly written in decimal while they should have been written in hexadecimal (oops!). * It modifies all the baremetal main functions (aka reset handlers) to exit with `exit(0)` instead of `abort()`. * It changes `syscall.Exit` to call `exit(code)` instead of `abort()` on baremetal targets. * It adds these new exit functions where necessary, implemented in a way that signals the correct exit status if running under QEMU. All in all, this means that `tinygo test` doesn't have to look at the output of a test to determine the outcome. It can simply look at the exit code.
73 строки
1,1 КиБ
Go
73 строки
1,1 КиБ
Go
// +build cortexm,qemu
|
|
|
|
package runtime
|
|
|
|
// This file implements the Stellaris LM3S6965 Cortex-M3 chip as implemented by
|
|
// QEMU.
|
|
|
|
import (
|
|
"device/arm"
|
|
"runtime/volatile"
|
|
"unsafe"
|
|
)
|
|
|
|
type timeUnit int64
|
|
|
|
var timestamp timeUnit
|
|
|
|
func postinit() {}
|
|
|
|
//export Reset_Handler
|
|
func main() {
|
|
preinit()
|
|
run()
|
|
|
|
// Signal successful exit.
|
|
exit(0)
|
|
}
|
|
|
|
func ticksToNanoseconds(ticks timeUnit) int64 {
|
|
return int64(ticks)
|
|
}
|
|
|
|
func nanosecondsToTicks(ns int64) timeUnit {
|
|
return timeUnit(ns)
|
|
}
|
|
|
|
func sleepTicks(d timeUnit) {
|
|
// TODO: actually sleep here for the given time.
|
|
timestamp += d
|
|
}
|
|
|
|
func ticks() timeUnit {
|
|
return timestamp
|
|
}
|
|
|
|
// UART0 output register.
|
|
var stdoutWrite = (*volatile.Register8)(unsafe.Pointer(uintptr(0x4000c000)))
|
|
|
|
func putchar(c byte) {
|
|
stdoutWrite.Set(uint8(c))
|
|
}
|
|
|
|
func waitForEvents() {
|
|
arm.Asm("wfe")
|
|
}
|
|
|
|
func abort() {
|
|
exit(1)
|
|
}
|
|
|
|
func exit(code int) {
|
|
// Exit QEMU.
|
|
if code == 0 {
|
|
arm.SemihostingCall(arm.SemihostingReportException, arm.SemihostingApplicationExit)
|
|
} else {
|
|
arm.SemihostingCall(arm.SemihostingReportException, arm.SemihostingRunTimeErrorUnknown)
|
|
}
|
|
|
|
// Lock up forever (should be unreachable).
|
|
for {
|
|
arm.Asm("wfi")
|
|
}
|
|
}
|